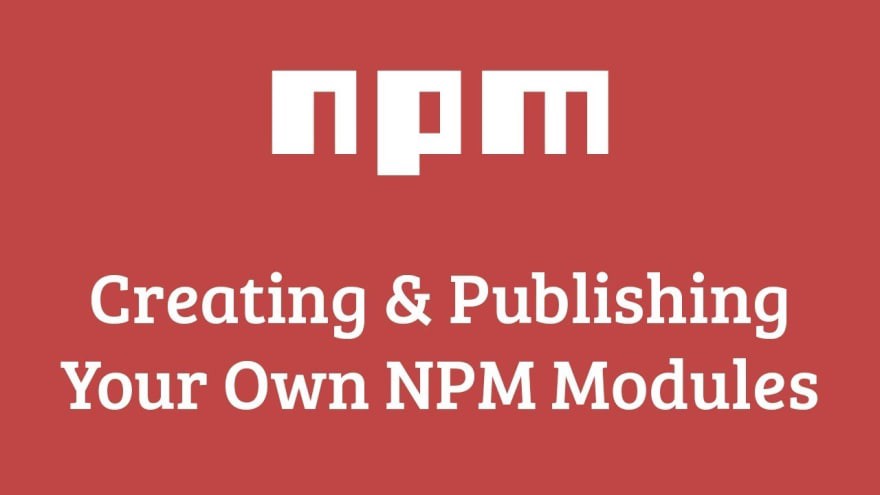
This article teachs you how to build and publish your own NPM (Node Package Manager) module to NPM registry (www.npmjs.com). It’s not difficult as you might think, as you know NPM module is just a collection of javascript functions and I’m going to write a module which can generates and returns random alphanumeric characters of any length (it seems very easy, right?). Hopefully at the end of this article, you’ll learn even more than you expected.
Before writing our module, make sure you already installed Node and Git (also create your account in GitHub) into your machine. You can use Bitbucket instead of GitHub but in my article I’m using GitHub.
Please search on Google and install them properly. I don’t want to guide you all things so let make yourself a little bit busy.
Firstly, let’s create a new project and we’re going to push it to GitHub and publish our module to NPM registry. I’ll create a new folder in my computer calls random-string-module and setup everything we need inside it (you can change the folder’s name with any name you want). Open your terminal or command prompt (in Windows) and going to this folder using cd command. The first thing I want to do is to initialize Git for our project, type
git init
to initialize empty Git repository. The next thing we’re going to do is going to GitHub and create our new repository with your GitHub account. I’ll let you do it by yourself so beware how you do and good luck 🙂
After creating your new repository on GitHub, open your project folder (random-string-module) using your text editor (Sublime Text, Atom, VSCode, …). I’m going to initialize our project using npm command, type
npm init
and fill all your project information (name, version, entry point, description, keywords, author, …) correctly. There are some important fields which you need to care about including name (make sure your name is unique to other existing packages), version (you need to understand your version pattern) and entry point especially the entry point which point to the javascript file that will be invoked when consumers of your module require it and it should be named as app.js but in my project I called it server.js. After filling all information, the command will create a package.json file with the content looks like this
Now I put our main logic inside my server.js file and export my function to others if there is anyone wants to use it. In the block of codes below I’m exposing the object which contains our random string function RandomChar and its purpose is to random a string includes characters from a to z and 0 to 9 with the length given by users.
module.exports = { RandomChar: function(num) { var string = "abcdefghijklmnopqrstuvwxyz0123456789"; var str = ''; var i = 0; while (i < num) { str += string.charAt(Math.floor(Math.random() * string.length)); i++; } return str; } }
Our hardest part of work is done now. I’ll create 2 new files .gitignore and README.md file before pushing all my codes to GitHub. If you don’t want to push unnecessary files to GitHub please put them in .gitignore file (e.g. node_modules folder, log files). README.md file is also important because it shows the instructions for users how to install and use our module so it must be readable and understandable (you can refer to my README.md file in my sample project attached to this article).
Finally, the rest of things we have to do is to publish our module to NPM registry. To do that we need to go to npmjs.com page and register your NPM account, it’s very very easy. After having your NPM account, open your terminal and log in into NPM using command
npm login
When you’ve already logged in, type
npm publish
to publish your module to NPM registry, that’s all the things you just have to do.
Now if you search random-string-module in npmjs page, it will display our module in the result list for other people to download.
Congratulation! You can download your own package into your machine and test it by yourself. Please fix any bugs if it has 😉
Source code link https://github.com/expedienthead/random-string-module